npx @teambit/bvm install
Setup micro frontend using Bit
Install bit
First create a workspace that contains env. We will be using bit with vue.js for the setup. It can be used with other frontend libraries as well.
bit new vue my-workspace --env teambit.vue/vue --default-scope my-org.my-scope
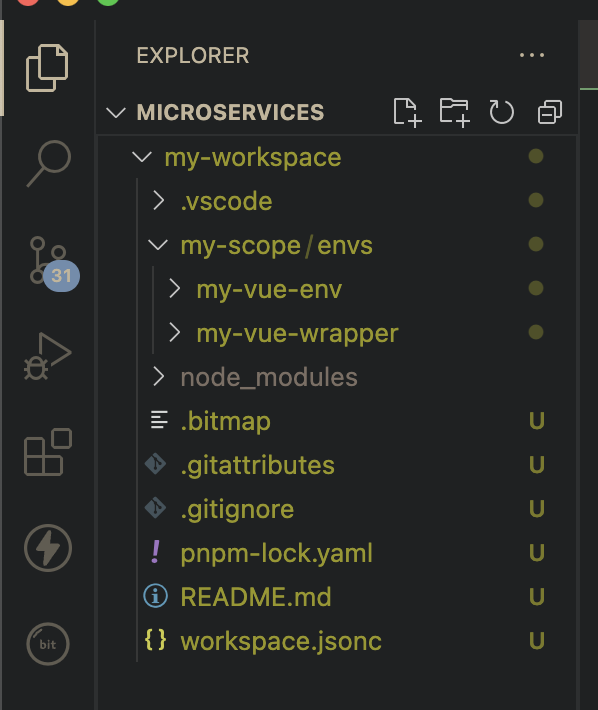
The above setup initialises the workspace. To know more about Bit workspace click here.
Components can be created using templates, as of now bit has 4 vue templates types.
Run the command to check the available templates.
bit templates
All available vue templates
- vue (A vue component template. Can be used for Vue components.)
- vue-composable (A vue composable template. Can be used for Vue composables.)
- vue-app (A vue application template. Can be used for Vue applications.)
- vue-env (A vue env template. Can be used for creating your own Vue env.)
Lets create vue application with name my-app and start the application.
bit create vue-app apps/my-app
bit use apps/my-app // this makes the app lodable in workspace
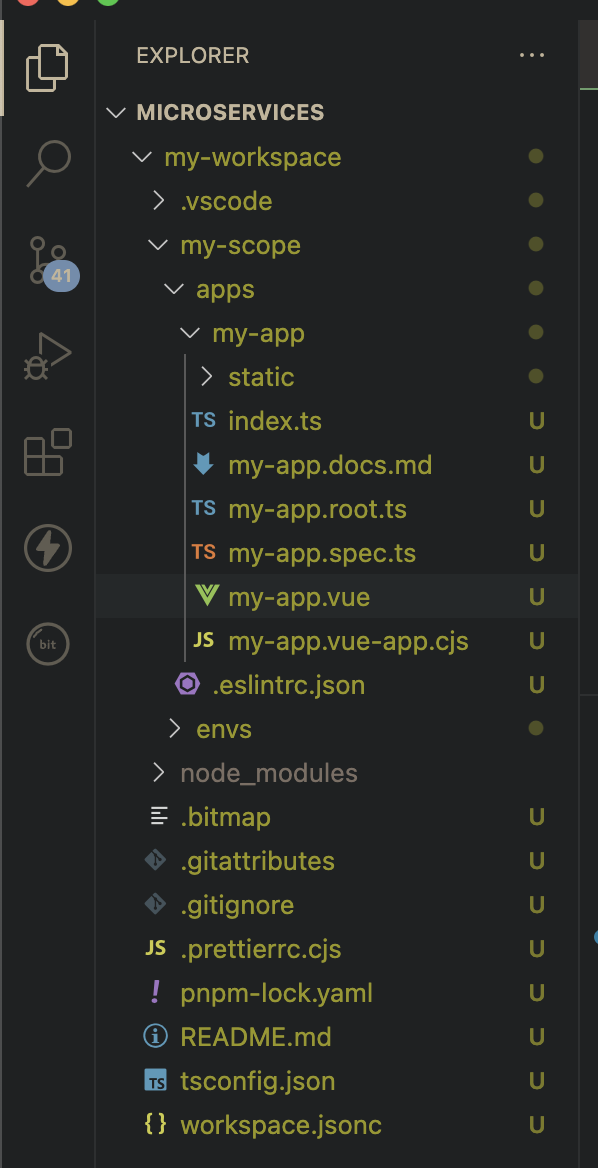
Start the application using following command
bit run my-app-app
Application name my-app-app can be changed by changing the name property value stored in my-app.vue-app.cjs.
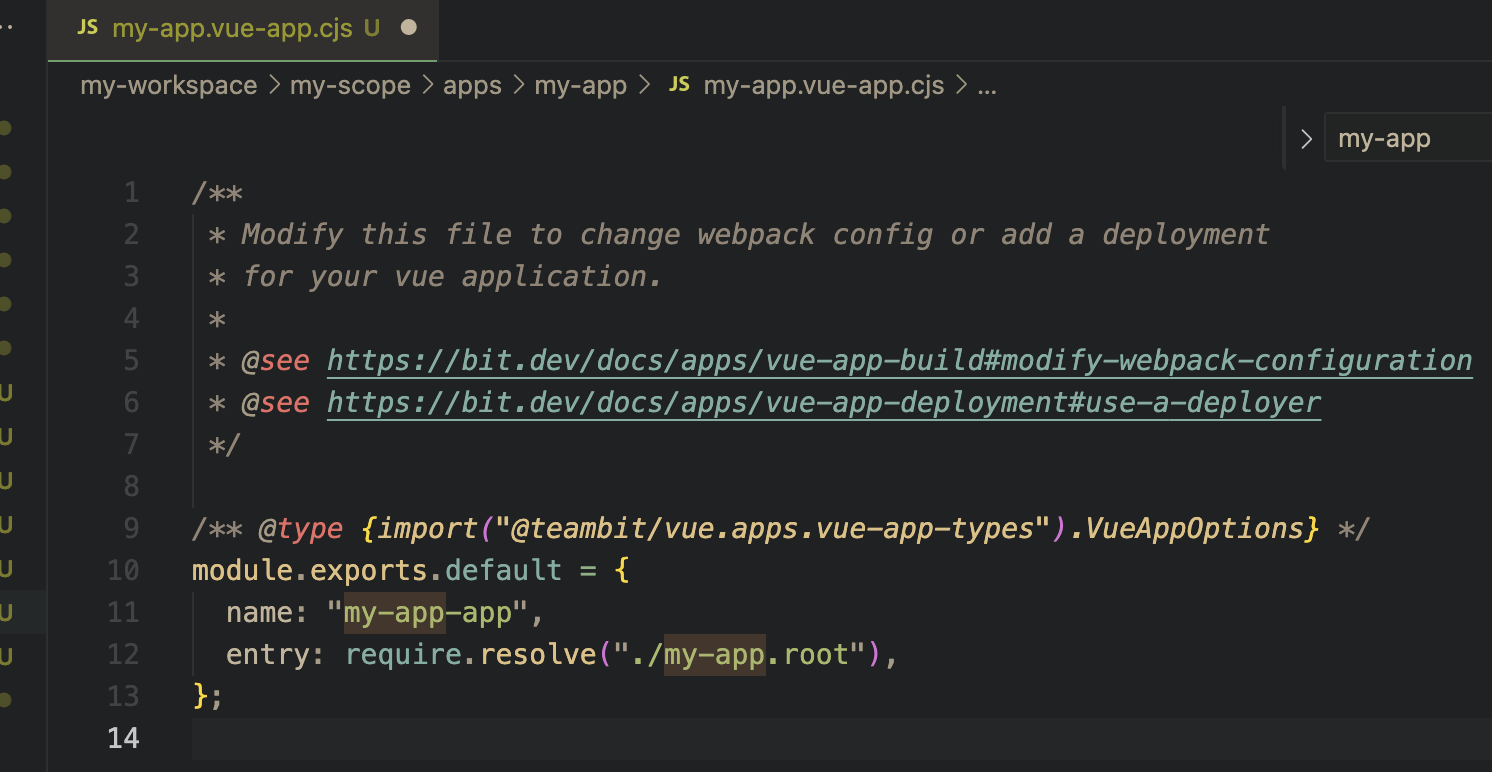
When you run the application you can see a counter present on screen. This is the default code present in the template. Delete the unwanted code and library vue-router to the application.
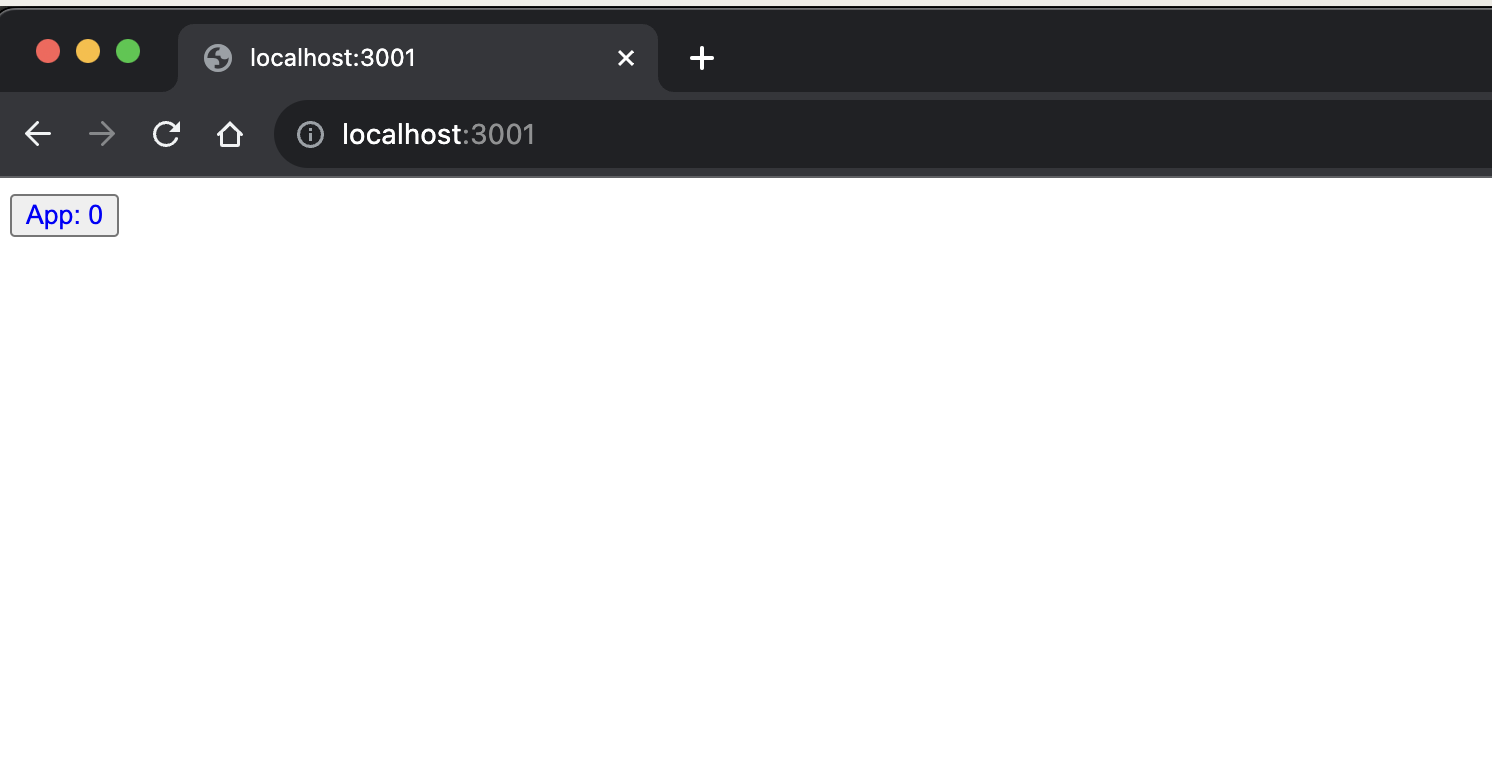
Run the following command to install vue-router and setup vue router in my-app.
bit deps set apps/my-app "vue-router"
I have setup two routes / and /about. After setup is complete both routes should work properly.
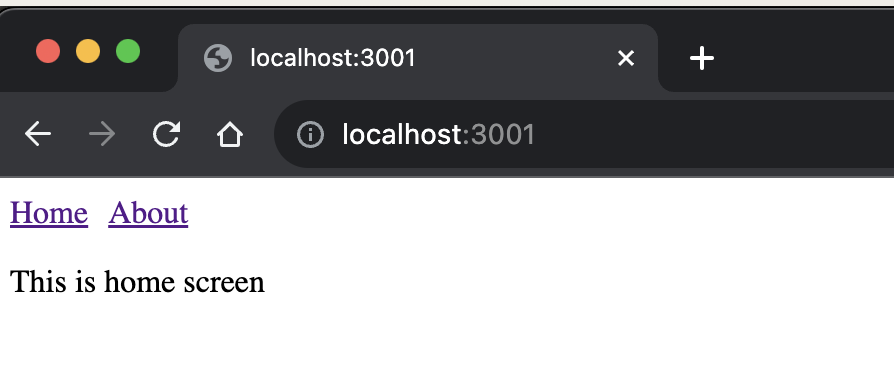
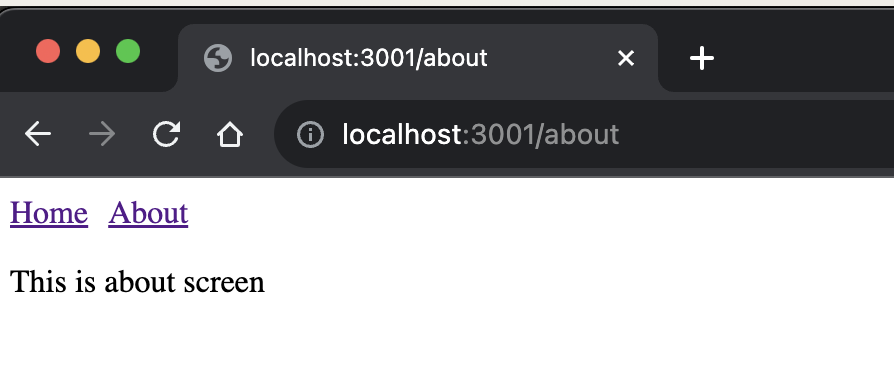
Routing is successfully setup if you are able to navigate on different routes.
Create common state management for all apps.
Add pinia as dependency for common/store.
Install pinia
bit create vue-composable common/store
bit deps set common/store "pinia"
bit install
After successfull installation setup pinia.
- Go to store.ts in common/store delete all the code from this file and paste below code. This will provide pinia instance to all the applications.
import { createPinia } from "pinia";
export const pinia = createPinia();
- To setup pinia in application go to the apps/my-app/my-app.root.ts and add the following code.
import { createApp } from "vue";
import App from "./my-app.vue";
import router from './router'
import {pinia} from "@my-org/my-scope.common.store";
const app = createApp(App)
app.use(pinia)
app.use(router)
app.mount("#app");
- Add routing to the main vue file, go to my-app.vue file and paste the following code.
<script setup>
import { RouterLink, RouterView } from 'vue-router'
</script>
<template>
<header>
<nav>
<RouterLink style="margin-right: 10px;" to="/">Home</RouterLink>
<RouterLink to="/about">About</RouterLink>
</nav>
</header>
<RouterView />
</template>
- Create a counter store in common/store/counter.js
import { defineStore } from "pinia";
import { ref } from "vue";
export const useCounter = defineStore("counter", () => {
const count = ref(0);
function inc() {
count.value++;
}
return { count, inc };
});
- Import the store in any app file to test updating values in store, I will import it in apps/my-app/views/HomeView.vue
<script setup>
import { useCounter } from '@my-org/my-scope.common.store/counter'
const counter = useCounter()
</script>
<template>
{{counter.count}}
<button @click="counter.inc">click</button>
<div>
<p>This is home screen {{count}} {{name}}</p>
</div>
</template>
- After successfull import start the project.
bit run my-app-app
- You will see a screen like this. if the value displayed is reactive when clicked on button you have successfully setup pinia in your app.
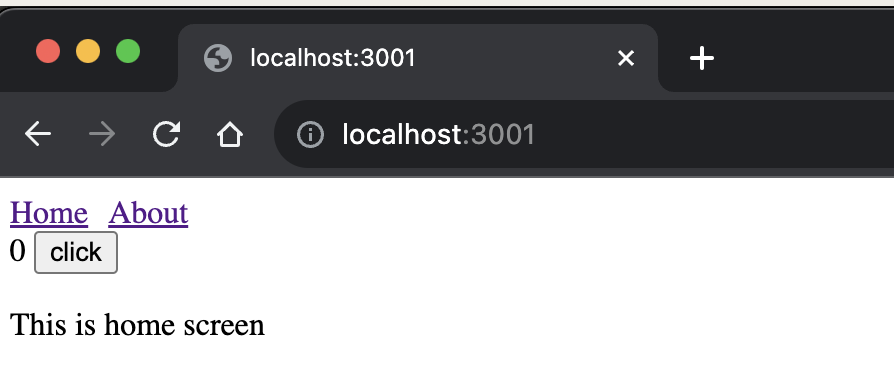
- Create another vue application, setup pinia in it and run the application. A common store being accessed by two applications.
bit create vue-app apps/my-task
bit use apps/my-task
apps/my-task/my-task.root.ts
import { createApp } from "vue";
import App from "./my-task.vue";
import { pinia } from "@my-org/my-scope.common.store";
const app = createApp(App);
app.use(pinia);
app.mount("#app");
apps/my-task/my-task.vue
<script setup>
import { useCounter } from '@my-org/my-scope.common.store/counter'
const counter = useCounter()
</script>
<template>
{{counter.count}}
<button @click="counter.inc">click</button>
<div>
<p>This is task screen {{count}} {{name}}</p>
</div>
</template>
run the application
bit run my-task-app
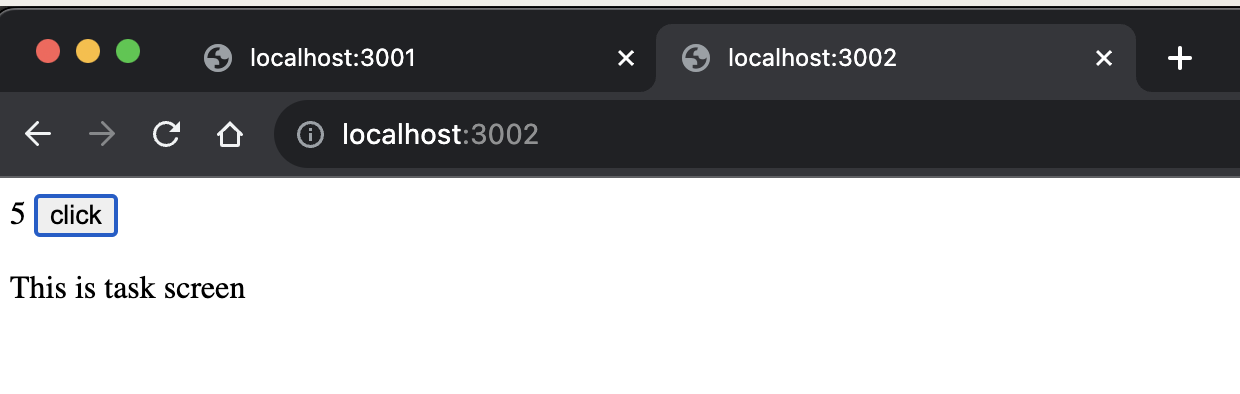
One common store is shared between two different applications my-app and my-task.
Link to code